Skipify Embedded Components
Learn how to integrate the Embedded Components SDK
Overview
Embedded Components are a flexible way to integrate the Skipify Commerce Identity Cloud solution by offering a modular integration design.
The Embedded Components SDK provides prebuilt components, while letting Skipify take care of shopper authentication, presenting payment information, and helping orchestrate payment submission and more.
Design where you want to embed prebuilt UI components to customize your checkout flow, or to further enhance shopper journeys on your website.
This section requires you to have set up your merchant account
Haven't set that up yet? No worries! Reach out to your friendly implementation engineer and they'll make sure your account is properly set up.
Embedded Components SDK - Overview
High level implementation steps:
- Look up shopper in our network using
shopper lookup
function - Display the
Authentication Component
to initiate and complete authentication of shoppers. - Display the
Payment Carousel Component
to display payment information for successfully authenticated shoppers. - Once checkout is finalized, use the selected payment method information to
submit the payment
.
Loading the Skipify Embedded Components SDK
To get started, you can load the SDK using:
<!-- TEST -->
<script src="https://stagecdn.skipify.com/sdk/components-sdk.js"></script>
<!-- LIVE -->
<script src="https://cdn.skipify.com/sdk/components-sdk.js"></script>
Initializing the Skipify Embedded Components SDK
Once you have completed the installation using one of the methods above, you can initialize the Skipify client SDK using:
const skipifyComponentsSdk = new window.skipify({
merchantId // your Skipify merchant id
});
Embedded Components SDK Technical Flow
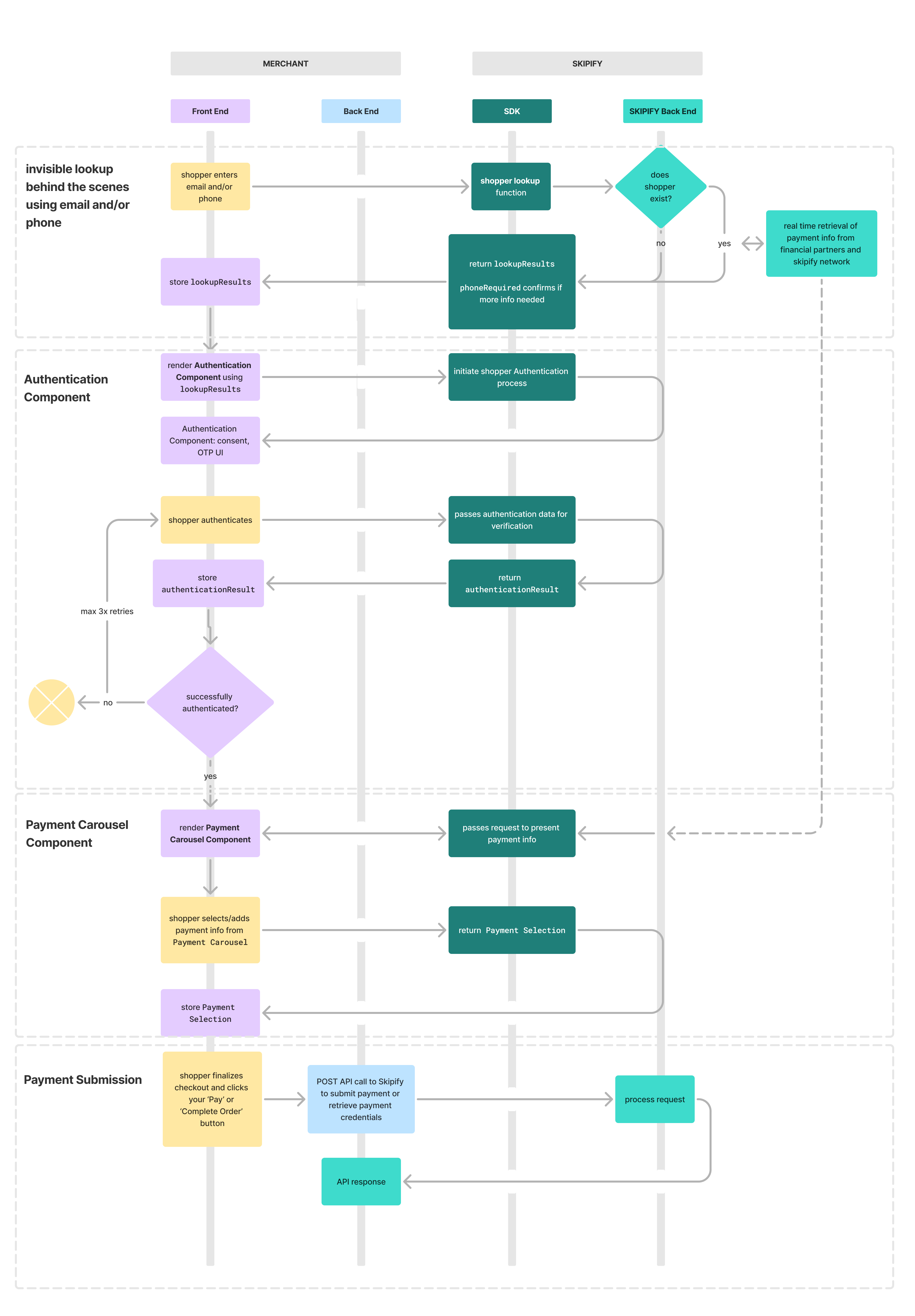
Theming
Skipify allows merchants to customize the appearance of the Authentication Component
and Payment Carousel Component
via a config
object in options. The available options are:
theme
: sets the component's color themefontFamily
: determines the font stylefontSize
: determines the font theme size
config: { // optional
theme: "light", // default theme or "dark"
fontFamily: "default", // default font family is poppins, or "serif", "sans-serif"
fontSize: "medium", // default font size or "small" or "large"
inputFieldSize: "medium" // default input field size or "small"
}
Shopper look up function
You can implement the lookup function using either:
- Embedded Components SDK lookup function
- API Only Flow's
POST /shoppers/lookup
The SDK's lookup
function will confirm if shoppers are recognized or in-network. This occurs seamlessly behind the scenes and will not impact your UI flow.
Request shopper lookup using either options below:
- shopper identifiers passed from your checkout UI to the
lookup
function. Shopper recognition results are passed back vialookupResults
. - the shopper's
deviceId
as detected by the SDK. Omit theshopper
object in the lookup function. Recognized shopper results are passed back vialookupResults
, and anerror
message will be returned for unrecognized shoppers.
If phone was not originally provided but lookupResults
requests it:
- collect
phone
in your UI and pass the data upon rendering theAuthentication Component
or, - let the
Authentication Component
prompt the shopper forphone
collection
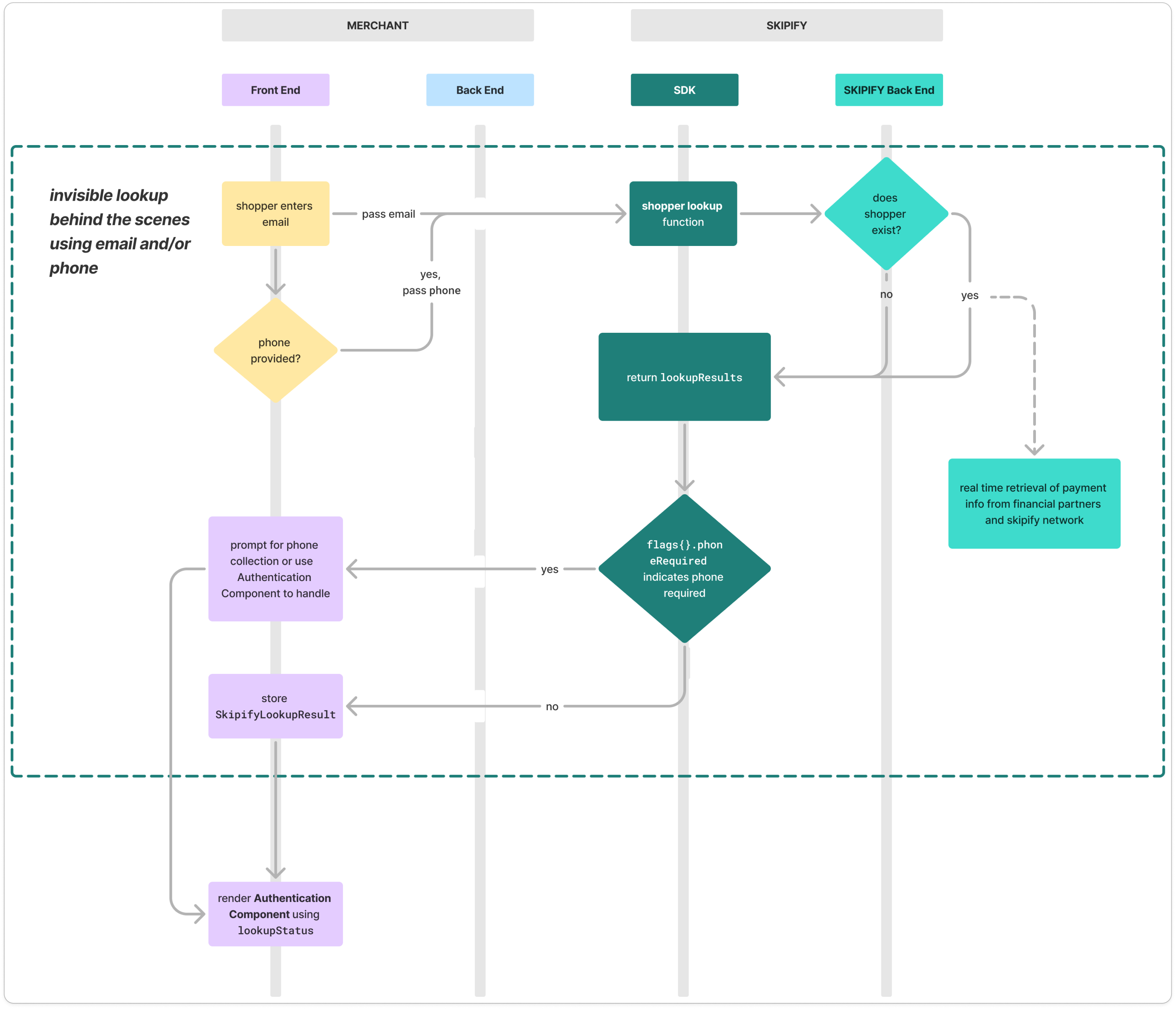
// send the shopper object to request look up using shopper identifiers
const shopper = {
email: '', // get your email from an input field or from logged-in shopper information
phone: '', // optional
};
// alternatively, omit the shopper object entirely to request look up using the shopper's deviceId detected by the Skipify Embedded Components SDK
skipifySdk.lookup(shopper).then((SkipifyLookupResults) => {
// store lookupResults object to use in later functions
});
// if using lookup with the shopper object, the lookupResults object is always returned
{
"challengeId": "7dc412fe-54b3-4f18-8985-8a7653ead125",
"flags": {
"potentialPaymentMethods": true, // indicates shopper may have payment methods with our financial partners or saved with Skipify
"phoneRequired": false,
"partnerProvidedPhone": false
},
"metadata": {
"maskedEmail": "r***@s***.com",
"maskedPhone": "6866"
},
"defaults": { // default authentication challenge channel
"destination": "phone",
"maskedChannel": "6866"
}
}
// if using lookup with deviceId, the lookupResults object is returned only if the shopper's device is recognized. If not recognized, an error message is returned
{
"error": {
"message": "Shopper not found"
}
}
The next step is to render theAuthentication Component
. If the shopper is unrecognized from shopper lookup (potentialPaymentMethods
is false) or deviceId
lookup (lookupResults
error
), you can continue and the shopper will be prompted to enter their payment details manually.
Authentication Component
The Authentication Component
handles shopper authentication process.
Render the Authentication Component
at a specified location using the prior lookupResults
. Alternatively, you can render the Payment Carousel Component
to handle both authentication and payment info presentation in the same location.
Use the displayMode
parameter to configure whether the Authentication Component should be embedded
or overlay
. An input field must be used for the overlay
option.
The SDK presents the shopper with a consent UI
to accept the authentication request. Use the optional sendOtp
boolean to streamline the experience for returning Skipify shoppers:
true
: bypass theconsent UI
for returning Skipify shoppers and skip straight to theOTP UI
false
: show theconsent UI
for all shoppers
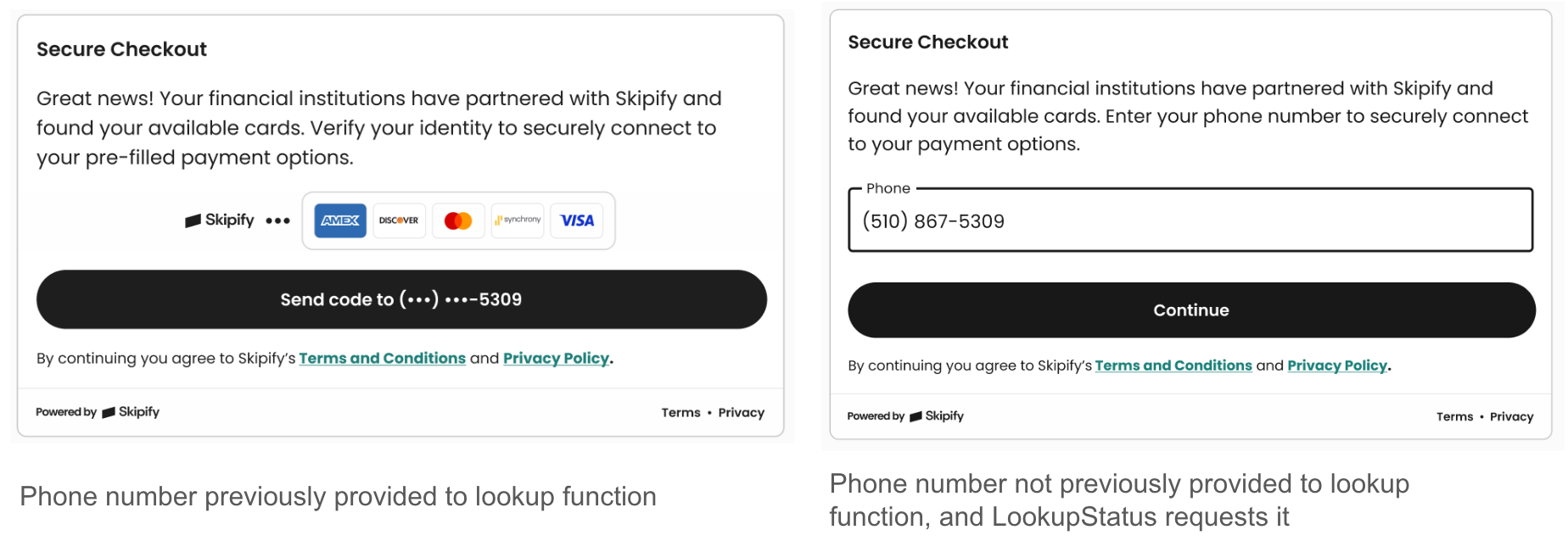
Authentication Component - consent UI
Once the shopper accepts the consent request, Skipify initiates the authentication process (currently OTP). Shopper inputs the necessary information in the UI to complete authentication.
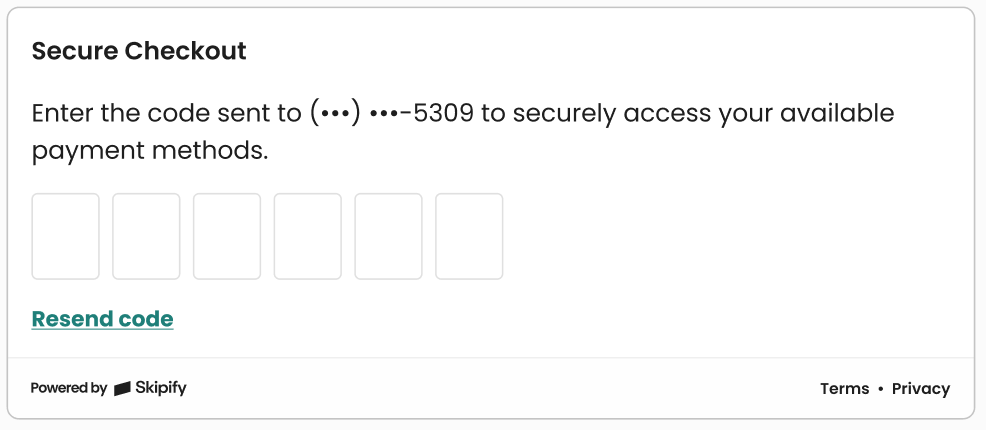
Authentication Component - OTP UI
The SDK triggers the appropriate callback to confirm if the shopper was successfully authenticated. authenticationResult
is passed back if successful.
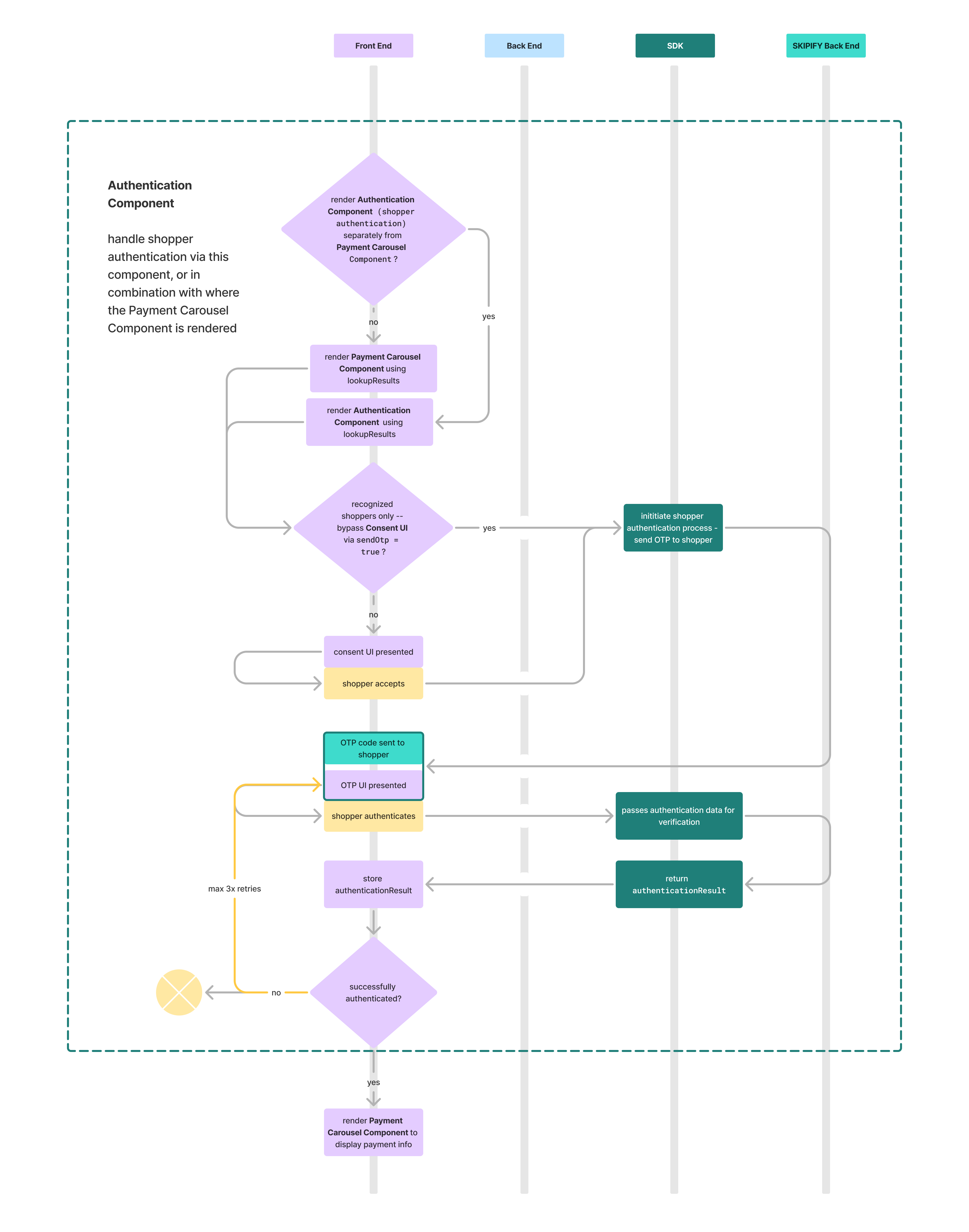
const options = {
onSuccess: (authenticationResult) => {
// store auth result
},
onError: (error) => {
// handle your error flow
},
phone: '', // optional to send, unless lookupResults flags{}.phoneRequired = true
sendOtp: boolean, // direct returning Skipify shoppers to OTP screen, bypassing the consent screen. This will auto trigger an authentication challenge sent to the shopper upon rendering the component
displayMode: "embedded", // or "overlay" the Authentication Component
config: { // optional
theme: "light",
fontFamily: "default",
fontSize: "medium",
}
};
// container to render in
const container = document.getElementById("my-container-id");
// render the Authentication Component
skipifySdk.authentication(lookupResults, options),render(container); // note, must be an input field for display mode "overlay"
{
"shopperId": "87b2e945-230a-49cc-a8b7-fd4e042b6385",
"sessionId": "991bac4b-6f0b-4fa5-912a-c1317497d480"
}
{
"challengeId": "7dc412fe-54b3-4f18-8985-8a7653ead125",
"flags": {
"potentialPaymentMethods": true,
"phoneRequired": false,
"partnerProvidedPhone": false
},
"metadata": {
"maskedEmail": "r***@s***.com",
"maskedPhone": "6866"
},
"defaults": {
"destination": "phone",
"maskedChannel": "6866"
}
}
Payment Carousel Component
The Payment Carousel Component
offers the following functionalities:
- auto filling and presenting the shopper's payment information. This includes cards from in-network financial partners and/or cards the shopper may have manually added to their Skipify account
- ability for shoppers to add/edit/delete manually added payment methods and info
- ability for the shopper to select which payment method they would like to use for checkout
- presenting and handling additional step-ups that the financial partner may require
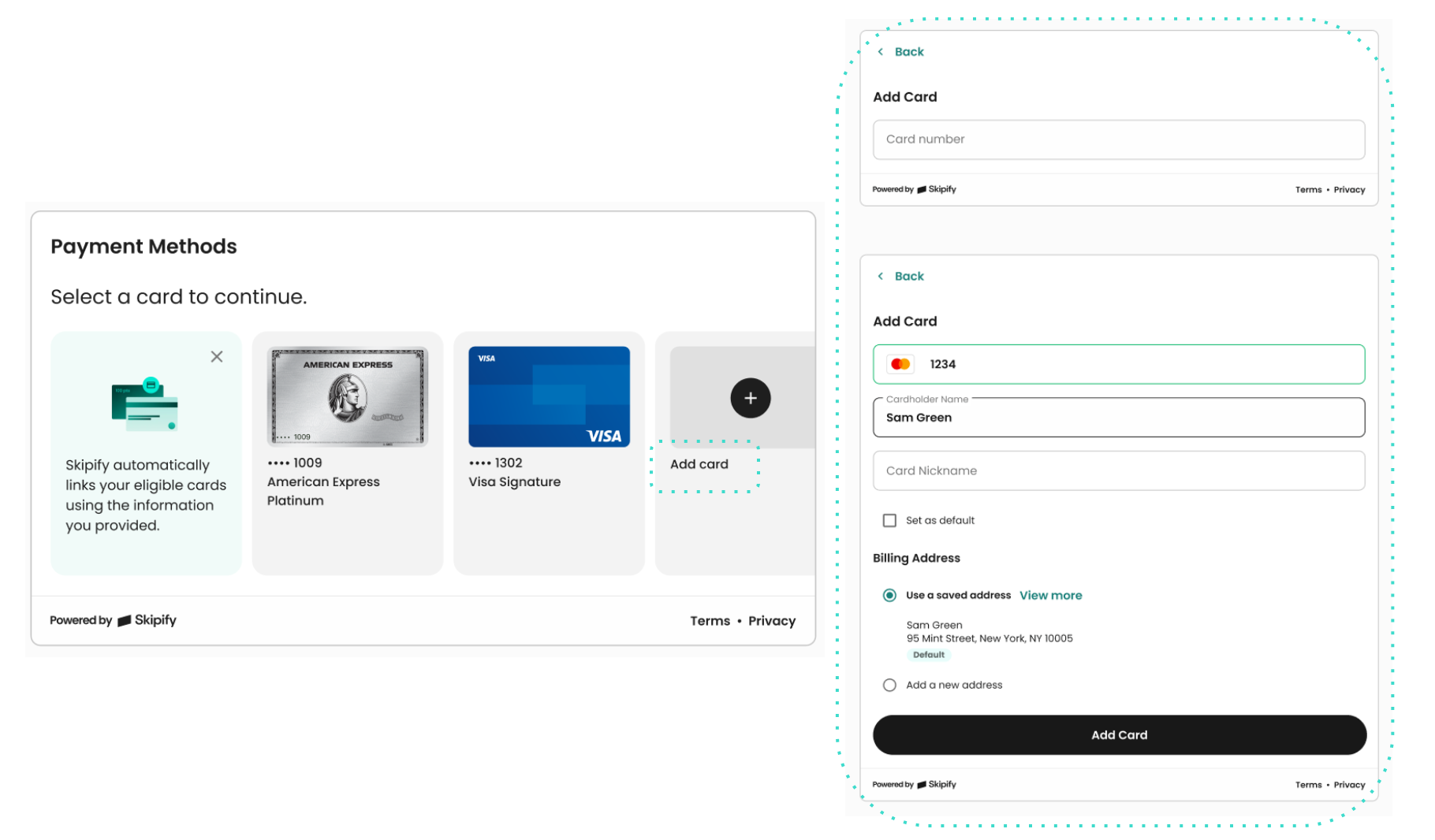
Payment Carousel Component
Render the Payment Carousel Component
using either:
lookupResults
if you would like to handle the authentication process and payment info presentation in the same stepauthenticationResult
if the shopper has already been successfully authenticated
The payment method that the shopper selects to use for checkout is summarized in PaymentSelection
. Use this information to submit the payment in the final step.
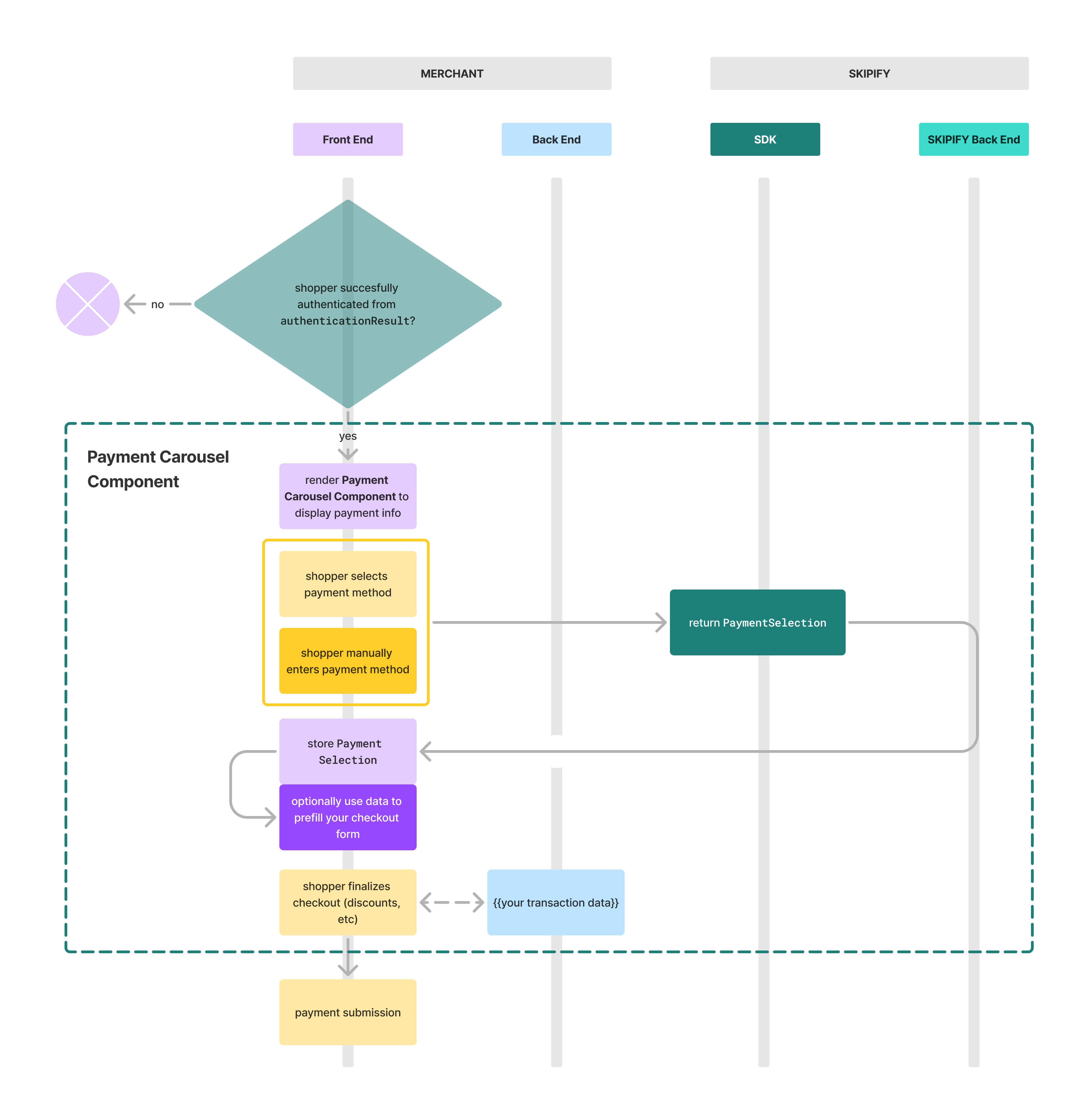
const options = {
onSelect: (result: PaymentSelection) => {
// the shopper's selected payment info for checkout
},
onError: (error) => {
// handle your error flow
},
amount: 80, // your order total
phone: "", // optional
config: { // optional
theme: "light", // default theme or "dark"
fontFamily: "default", // default font family is poppins, or "serif", "sans-serif"
fontSize: "medium", // default font size or "small" or "large"
inputFieldSize: "medium" // default input field size or "small"
}
};
// render the Payment Carousel Component at a specified location
const carouselContainer = document.getElementById("merchant-carousel-container");
// render the Payment Carousel Component based on auth results or lookup results
if(authenticationResult){
skipifySdk.carousel(authenticationResult, options).render(carouselContainer);
},
else{ // shopper not authenticated using lookup results instead
skipifySdk.carousel(lookupResults, options).render(carouselContainer);
}
PaymentSelection = {
paymentId: "8420a18b-3245-4694-b2fd-e8f3b99bf360",
sessionId: "991bac4b-6f0b-4fa5-912a-c1317497d480",
address: {
address1: "77 E 4th St",
city: "New York",
country: "US",
firstName: "Jane",
lastName: "Doe",
phoneNumber: "2123580650",
state: "NY",
zipCode: "10003",
},
metadata: { // payment method metadata - card example below
networkType: "Amex",
expiryDate: "05/26",
lastFour: "4739",
};
};
If the shopper's session times out (30min), the Payment Carousel
Component will request authentication again.
Payment Submission
Skipify provides flexibility in how you process payments, allowing you to choose between two authorization methods:
Skipify authorizes on your behalf
The default flow is for Skipify to process the transaction on your behalf.
Once the shopper selects a payment method and finalizes the checkout, use the /payments
end point to send the payment request. Skipify will authorize the transaction by connecting to your PSP and return the results synchronously.
Call POST /payments
to submit the payment request
Staging:
POST https://services.staging.skipify.com/payments
Production:
POST https://services.skipify.com/payments
This endpoint uses API Key Authentication
Check out our API KEY Authentication Section to learn more
POST /payments
{
"paymentId": "73c335b2-0dad-4e4a-b10b-950614f80953",
"sessionId": "ae5686ad-bcc0-425a-bc84-7e9a142b61d3",
"amount": 5000,
"merchantReference": "YOUR MERCHANT REFERENCE HERE",
"enableRecurring": false
}
{
"transactionId": "73c335b2-0dad-3p3a-b10b-950614f80953",
"pspTransactionId": "dE3Hnj7c_SD9",
"status": "Authorized",
"amount": 5000,
"initialAmount": 5000,
"pspRawResponse": "FWWp",
"completedAt": "2025-07-21T17:32:28Z",
"isPartial": false,
"paymentMethod": {
"details": {
"lastFour": "0005",
"networkType": "visa",
"cardArtUrl": "https://example.com/card-art.png"
}
}
}
{
"code": "SPECIFIC_ERROR_CODE",
"message": "Something went wrong, here is what happened."
}
Request Body Parameters
POST /v1/payments
Parameter Name | Required? | Type | Description |
---|---|---|---|
paymentId | yes | string | This is the paymentId received from the shopper's payment carousel selection. |
sessionId | yes | string | This is the sessionId received from the shopper's payment carousel session. |
amount | yes | integer | The total amount for this purchase in cents (USD). |
merchantReference | yes | string | This is your unique merchant identifier/invoice number for this transaction. |
enableRecurring | no | boolean | Flag to indicate whether this payment request is for recurring payment |
Request Headers
In addition to the API Key Authentication headers, the below headers are also required:
Header Name | Description |
---|---|
X-Idempotency-Key | Unique identifier for the request. |
Response Body Parameters
Parameter Name | Type | Description |
---|---|---|
transactionId | string | The Skipify transaction identifier. |
pspTransactionId | string | The transactionId from the PSP. |
status | string | Allowed Values = Authorized, Captured, Failed. |
amount | integer | The amount, in cents in USD that was authorized or captured. |
initialAmount | string | The initial requested amount to Authorize. May not match 'amount'. |
completedAt | string - date/time | The timestamps in UTC that the transaction was completed |
lastFour | string | The last 4 digits of the card used to pay. |
networkType | string | The card brand of the card used to pay - i.e. - VISA |
pspRawResponse | string | The response from the PSP without any parsing or alteration. |
isPartial | boolean | Is the merchant has been configured to accept partial authorizations - this flag will be true when only a partial amount of the transaction was approved. |
recurringMethodId | string | Identifier for recurring payment method used for payment |
paymentMethod | object | Container object that holds payment method information |
paymentMethod.details | object | Nested object within paymentMethod containing specific payment card details |
paymentMethod.details.lastFour | string | The last 4 digits of the payment card used for the transaction |
paymentMethod.details.networkType | string | The card brand/network of the payment card - e.g. Visa, Mastercard, Amex, Discover |
paymentMethod.details.cardArtUrl | string | URL pointing to the card artwork/image associated with the payment method |
Retrieve payment credentials (network tokens or PAN) to authorize directly
Requires approval for and additional configurations
Reach out to your implementation engineer to ensure your use case is approved. Once approved, your merchantAccount will be configured to allow for Network Token and/or PAN retrieval.
Skipify provides the ability for merchants to submit authorizations themselves to offer true PSP agnostic support.
This flow consists of three key steps:
- Request Payment Credentials – Make an API call to Skipify to request the payment details (e.g., network token or PAN).
- Receive Credentials via Webhook – Skipify will send the requested credentials securely to your webhook.
- Confirm Authorization Response – After processing the payment externally, send the authorization response back to Skipify to finalize the transaction.
Call the /v1/payments/credentials
API to request payment credentials using the sessionId
and the shopper's selected payment method paymentId
Staging:
POST https://services.staging.skipify.com/payments/credentials
Production:
POST https://services.skipify.com/payments/credentials
This endpoint uses API Key Authentication
Check out our API KEY Authentication Section to learn more
// POST /payments/credentials
{
"paymentId": "73c335b2-0dad-4e4a-b10b-950614f80953",
"sessionId": "ae5686ad-bcc0-425a-bc84-7e9a142b61d3",
"merchantReference": "NjghZplGtIx3ap9ziC",
"amount": 5000,
"currencyCode": "USD"
}
{
"code": 202,
"message": "Accepted"
}
{
"code": "SPECIFIC_ERROR_CODE",
"message": "Something went wrong, here is what happened."
}
Request Body Parameters
POST /v1/payments/credentials
Parameter Name | Required? | Type | Description |
---|---|---|---|
paymentId | yes | string | This is the paymentId received from the shopper's payment carousel selection. |
sessionId | yes | string | This is the sessionId received from the shopper's payment carousel session. |
amount | yes | integer | The total amount for this purchase in cents (USD). Required when merchantAccount is setup for Network Tokens. |
currencyCode | yes | string | The currency of the payment (ISO 4217). Required when merchantAccount is setup for Network Tokens. |
merchantReference | yes | string | This is your unique merchant identifier/invoice number for this transaction. |
HTTP 202 Accepted response status will be returned as a successful acknowledgement in the POST /v1/payments/credentials
response.
Payment_Credentials Webhook
The actual payment credentials will be sent asynchronously to the URL configured on your merchant account.
// This JSON payload will be sent to your configured URL for the PAYMENT_CREDENTIAL webhook
{
"eventName": "PAYMENT_CREDENTIALS", // The Event that triggered the webhook
"paymentId": "26gug05e-dcd3-420e-be91-f0483724f74d", // The payment method selected by the shopper for checkout
"merchantId": "c1brc8b60-6dd4-4136-88e1-c9e5b670f1ca0", // The MerchantId that the event was triggered for
"merchantReference": "merchantUniqueReference", // Your unique order or cart id
"cardDetails": {
"networkType": "visa", //Card Brand
"networkTransactionId": "8rhjoefh9fdsf8h", //The Network TransactionId for this card
},
"credentials": [
{
"type": "NETWORK_TOKEN",
"cryptogram": "1566", // conditional - provided when avail dynamic data for transaction processing
"cvv": "123", // conditional - dynamic cvv to be used w/ Discover Network Token
"token": "0987654321098745", // Network token value
"expirationMonth": "12",
"expirationYear": "2028"
},
{
"type": "PAN",
"pan": "1234567890123456", // Credit card PAN
"expirationMonth": "12",
"expirationYear": "2028"
}
]
}
Submitting the PSP authorization to Skipify
Authorize the transaction directly. Then, submit the PSP authorization result to Skipify .
Staging:
POST https://services.staging.skipify.com/payments/external-response
Production:
POST https://services.skipify.com/payments/external-response
This endpoint uses API Key Authentication
Check out our API KEY Authentication Section to learn more
// POST /v1/payments/external-response
{
"paymentId": "73c335b2-0dad-4e4a-b10b-950614f80953",
"pspRawResponse": "SGVsbG8gV29ybGQ=", // "Hello World" base64 encoded
"pspHostname": "Stripe",
"amount": 5000,
"currencyCode": "USD",
"merchantReference": "merchantUniqueReference", // Your unique order or cart id
"success": true,
"isRecurring": true
}
No Content
{
"code": "SPECIFIC_ERROR_CODE",
"message": "Something went wrong, here is what happened."
}
Request Body Parameters
POST /v1/payments/external-response
Parameter Name | Required? | Type | Description |
---|---|---|---|
paymentId | yes | string | This is the paymentId received from the shopper's payment carousel selection. |
pspRawResponse | yes | string | This is a base64 encoded string representing the full unformatted response you received from your PSP when submitting the Authorization. |
pspHostname | yes | string | This is the name of your PSP - i.e. - Stripe |
success | yes | boolean | Indicates if the response from the PSP was a successful or failure response. |
amount | no | integer | The total amount for this purchase in cents. |
currencyCode | no | string | The currency of the payment (ISO 4217). Required when amount is provided. |
isRecurring | no | boolean | Flag to indicate whether this payment request was for a recurring payment (CardOnFile). Defaults to False. |
merchantReference | no | string | This is your unique merchant identifier/invoice number for this transaction. |
Updated about 3 hours ago