Skipify Checkout SDK
Learn how to integrate the Skipify Checkout SDK
Overview
The Email lookup and Skipify Button use the Skipify Checkout SDK for a range of features. This guide shows you how to set up the SDK on your website. The various features of the SDK are further enumerated in additional articles in this section.
This section requires you to have set up your merchant account
Haven't set that up yet? No worries! Reach out to your friendly implementation engineer and they'll make sure your account is properly set up.
- Set up your merchant account including relevant configurations for your use case
- Implement the Email Look up, Skipify Button via the Skipify Checkout SDK which includes synchronous callbacks
- Implement asynchronous webhooks
Checkout SDK Technical Flow - Summary Overview
High level Checkout SDK implementation flow
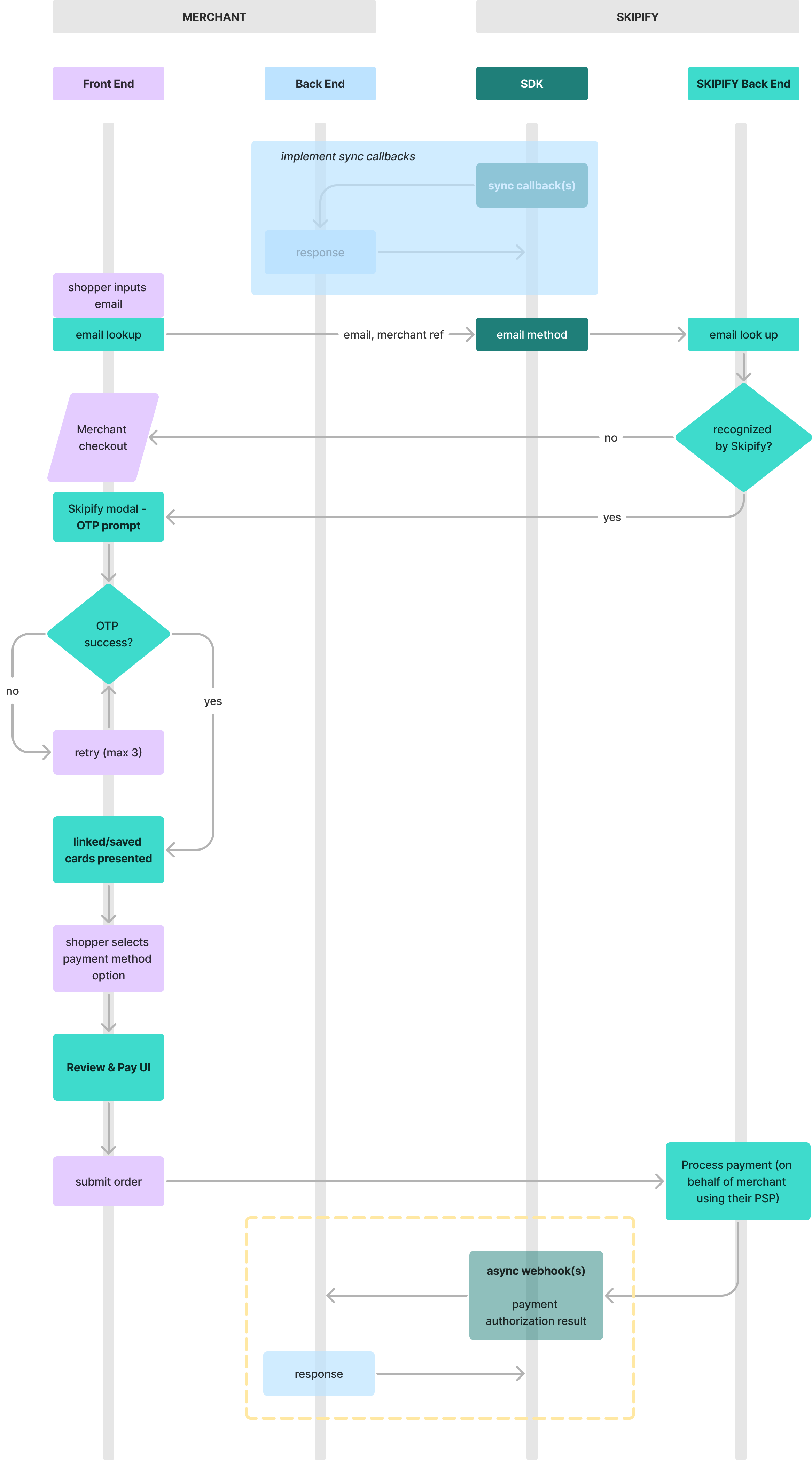
Requirements to use the Skipify Checkout SDK
Merchant Domains
Important
Provide relevant domains for testing in lower environments that will need to be whitelisted by your Skipify Implementation Engineer.
Localhost will need to be added for local development (ex.
https://localhost:5001
).
If you are using Ionic (iOS) add (capacitor://localhost
as your merchant domain).In Production, domains are added in the Skipify Merchant Portal.
Merchants are required to provide a set of allowed domains that Skipify uses to validate before initializing the Skipify Checkout SDK. Only configured domains will be able to leverage the Skipify Checkout SDK. You will need to provide that information to your implementation engineer during onboarding.
Loading the Skipify Checkout SDK
Skipify provides several options to integrate the Connected Checkout experience into your website. To get started, you can load the SDK using:
<!-- TEST -->
<script src="https://stagecdn.skipify.com/sdk/checkoutsdk.js"></script>
<!-- LIVE -->
<script src="https://cdn.skipify.com/sdk/checkoutsdk.js"></script>
$ npm install @skipifycheckout/checkout-sdk
$ yarn add @skipifycheckout/checkout-sdk
Initializing the Skipify Checkout SDK
Once you have completed the installation using one of the methods above, you can initialize the Skipify client SDK using:
const merchantId = "<your-merchant-id>";
let skipifyClient: any = null;
export const getSkipifyClient = () => {
if (skipifyClient) {
return skipifyClient;
} else {
skipifyClient = new window.skipify({
merchantId,
})
return skipifyClient;
}
}
import Skipify from '@skipifycheckout/checkout-sdk';
const skipifySdk = new Skipify({
merchantId: '<your-merchantId>',
});
Skipify Email Lookup
Email Lookup will launch the Connected Checkout experience for recognized shoppers using any email field on your checkout page.
skipifySdk.email("your_merchantReference").enable( < your_input_object > );
Methods
- The SDK
email
function is used to configure Email Lookup. PassmerchantReference
, your unique reference related to the order or cart. enable
is used to activate the Email Lookup functionality on your specified input field.
Skipify Button
Implement the Skipify Button anywhere on your website to accelerate checkout.
- Recognized shoppers are presented with their payment methods and checkout information.
- Shoppers new to the Skipify ecosystem have the option to save their info and payment methods for faster future checkouts anywhere that accepts Skipify.
skipifySdk.button("your_merchantReference").render( < your_container > );
const options = {
onClose: (myRef: string, success: boolean) => {
console.log('On close')
},
onApprove: (myRef: string, data: any) => {
console.log('On approve')
},
email: string,
phone: string
}
// Render Skipify button
skipifyClient.button("my-ref-test", options).render(buttonRef.current)
// Render Skipify button
const button = skipifyClient.button("my-ref-test", options)
button.render(buttonRef.current)
button.setOptions({phone: string, email: string})
Methods
button
is used to create the Skipify Button component instance. PassmerchantReference
, your unique reference related to the order or cart.render
is used to display the configured button on your website via the specified container. The button can render in any container element and the component will inherit the width of the container size.
Button Cobranding Configurations (optional)
Optional configurations available via the SDK in options
object:
textColor
: button text colorbgColor
: button colorbgHoverColor
: button color upon hovering over itlogoPlacement
: determines where the Skipify logo appears, 'inside' or 'below' the buttonbuttonLabel
: sets the button text either 'Buy Now' or 'Pay Now'
Prefill User Data in the Button Checkout Experience (optional)
Optional data accepted in options
object
email
: pass the shopper email to be prefilledphone
: pass the shopper phone to be prefilled
// Button options
const options = {
onClose: (myRef: string, success: boolean) => {
console.log('On close UI callback triggered')
},
onApprove: (myRef: string, data: any) => {
console.log('On approve UI callback triggered')
console.log(data)
},
// Button cobranding configs
textColor: '#00ff00',
bgColor: '#1f1f1f',
bgHoverColor: '#676767',
buttonLabel: "Buy Now",
// Add user data
email: email,
phone: phone,
}
// Render Skipify button
const skipifyButton = skipifyClient.button(merchantRef, options).render(buttonRef.current)
// Change Skipify button options
skipifyButton.setOptions(options)
SDK Technical Flow
Synchronous Callbacks
Before Skipify can submit a payment on your behalf, we need to gather some information about the order. Implement the applicable callbacks relevant to your business use case so that Skipify can retrieve info to facilitate a seamless shopper experience.
Skipify servers will send a request to your specified URL when needed. You will need to provide your implementation engineer a callback URL(s).
Overview
There are three callback events available via the SDK:
ORDER_RESOLVE
- defines amount per line items or cartORDER_CHANGE
- defines tax, shipping address, discountsORDER_VERIFY
- validation before payment processing
Callback Event Descriptions
Event | Implementation required | Response required | Description |
---|---|---|---|
ORDER_RESOLVE | yes | yes | Respond to this callback event to return further details about the cart. |
ORDER_CHANGE | no | yes | Implement this callback event to return additional information about the order as it relates to shipping, tax & discount adjustments, promos or any order quantity changes by your customer. |
ORDER_VERIFY | no | yes | Implement this callback event to confirm whether the information is accurate. This event is used to validate the order details before a payment is processed. |
Standard request payload for Callback Events
param | description |
---|---|
eventName | string Skipify event code name |
eventType | string sync |
live | boolean true or false |
payload{} | object merchantReference : string: Your unique reference related to the order or cart. This value will be passed to the PSP merchantID : string : Your Skipify merchant ID cart : object shippingAddress : object shippingOption : selected shipping option ID discountCode : discount code String metadata : object |
ORDER_RESOLVE Callback Event
This callback event will be used to render cart details about the order. Skipify will pass your unique merchantReference
you defined when you initiated the SDK.
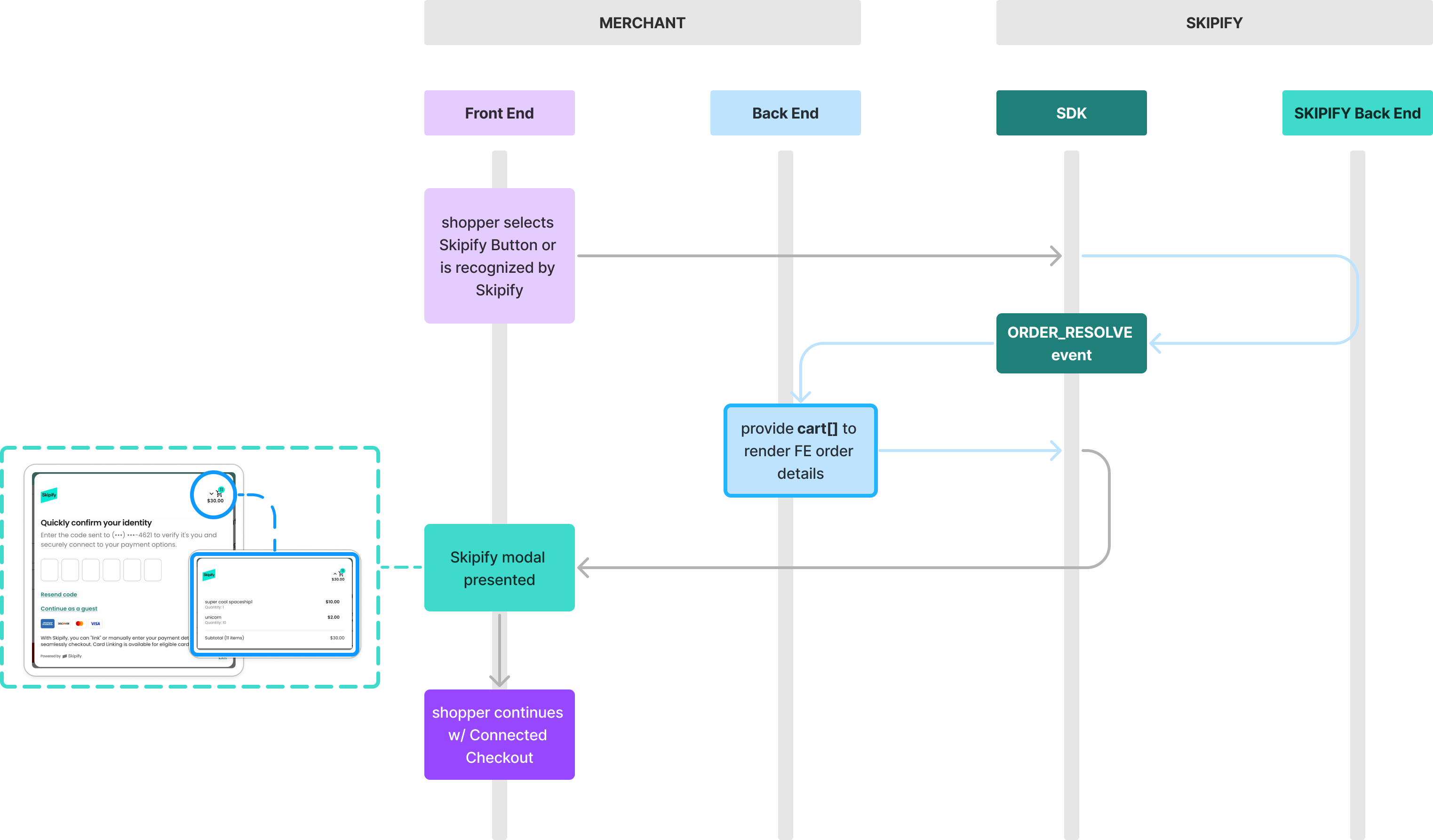
Sample ORDER_RESOLVE Request and Response
Please note the additional response parameters to include L2 and L3 fields *only supported with certain PSPs, check with your Implementation Engineer**
{
"eventName": "ORDER_RESOLVE",
"eventType": "sync",
"live": false, // this can be either true or false depending on the environment
"payload": {
"merchantReference": "YOUR_MERCHANT_REFERENCE", // this is your unique order or cart reference
"merchantId": "YOUR_MERCHANT_ID" // this is your skipify merchant ID
}
}
{
"cart": [
{
"itemId": "IT123123",
"title": "Line item one",
"quantity": 1, // integer
"productType": "physical",
"price": {
"value": 1050, // $10.50
"uom": "USD"
},
"image": {
"url": "URL HERE",
"alt": "text"
}
},
{
"itemId": "IT4435343",
"title": "line item 2",
"quantity": 2, // integer
"productType": "physical",
"price": {
"value": 2125, // $21.25
"uom": "USD"
},
"image": {
"url": "URL HERE",
"alt": "text"
}
}
]
}
{
"cart": [
{
"itemId": "IT123123",
"title": "Line item one",
"quantity": 1, // integer
"productType": "physical",
"description": "describe the item",
"price": {
"value": 1050, // $10.50
"uom": "USD"
},
"commodityCode": "com-code",
"productCode": "prod-code",
"enhancedSchemeData": {
"discountAmount": {
"value": 100, // $1.00
"uom": "USD"
},
"taxDetails":{
"value": 100, // $1.00
"uom": "USD"
},
"uom": "unit"
}
},
{
"itemId": "IT4435343",
"title": "line item 2",
"quantity": 2, // integer
"productType": "physical",
"description": "describe the item",
"price": {
"value": 1050, // $10.50
"uom": "USD"
},
"commodityCode": "com-code",
"productCode": "prod-code",
"enhancedSchemeData": {
"discountAmount": {
"value": 100, // $1.00
"uom": "USD"
},
"taxDetails": {
"value": 100, // $1.00
"uom": "USD"
},
"uom": "unit"
}
}
]
}
Request Parameters
For this event the request parameters are only the standard parameters
Response Parameters
Parameter Name | Type | Description |
---|---|---|
cart | object | This will be the cart items |
Cart Object Parameters
Parameter Name | Type | Required | Description |
---|---|---|---|
title | string | yes | The title of the item(s) to be displayed |
itemId | string | yes | This is the ID of your line item |
productType | string | no | The type of product. Example: shirt, pizza |
quantity | integer | yes | Line item quantity |
description | string | no | Describes the cart item |
price | unit object | yes | These are the amounts for each product / item |
image | price object | no | {url:"", alt:""} |
unit object Parameters
Parameter Name | Type | Required | Description |
---|---|---|---|
value | integer | yes | The value of an item in minor units. $20.50 would be sent as 2050. |
uom | string | yes | ISO4217 three letter code identifying the currency. Skipify is only processing transactions in USD at this time. |
image object Parameters
Parameter Name | Type | Required | Description |
---|---|---|---|
url | string | no | Url of the image |
alt | string | no | A text to be displayed on hover |
ORDER_CHANGE Callback Event
This callback event will be used to render more details and/or shopper-made updates about the order. Skipify will pass your unique merchantReference
you defined when you initiated the SDK along with other parameters below.
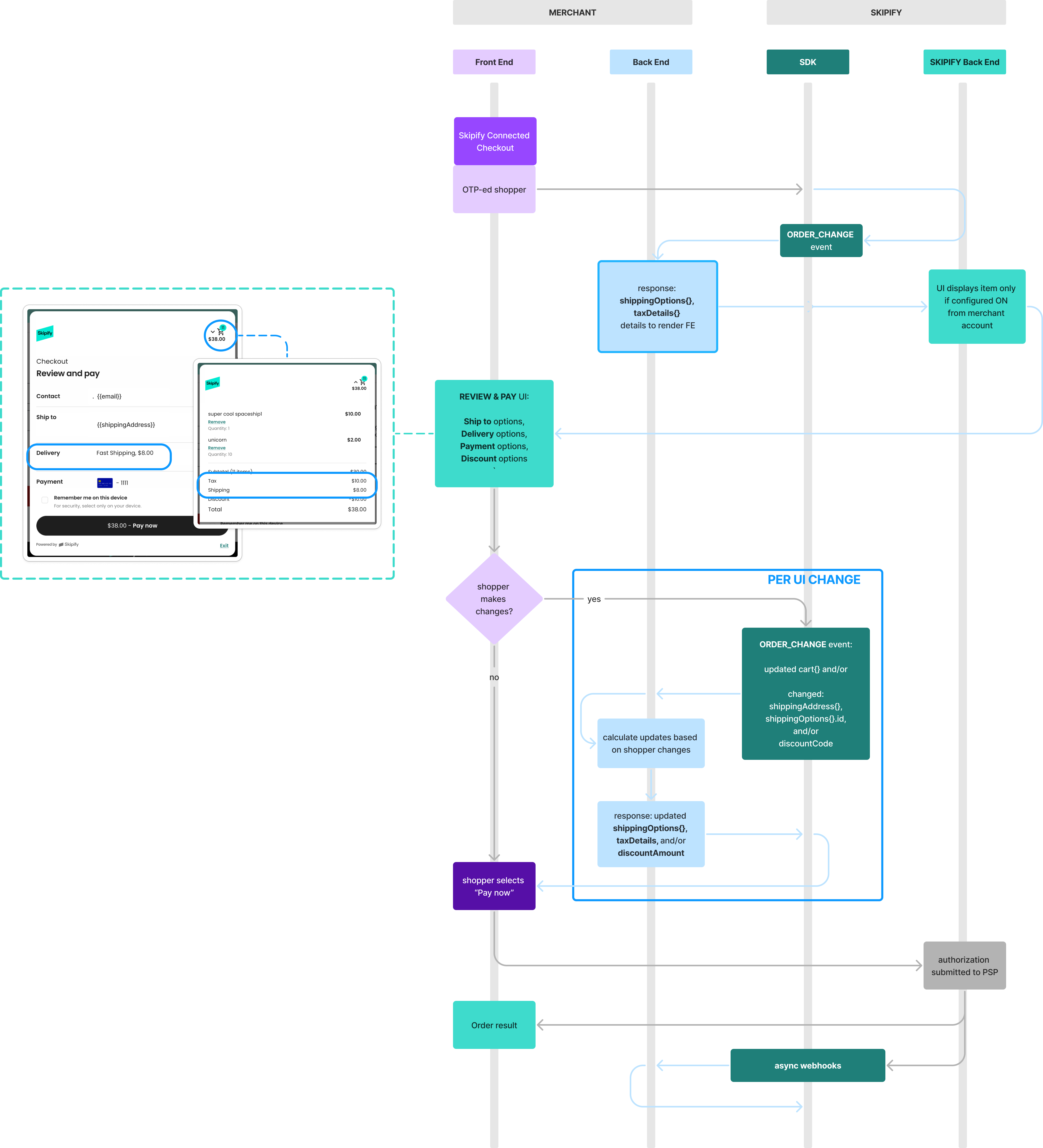
Sample ORDER_CHANGE Request and Response
{
"eventName": "ORDER_CHANGE",
"eventType": "sync",
"live": false,
"payload": {
"cart":[], // your cart details here
"shippingAddress": {
"firstName": "John",
"lastName": "Doe",
"address1": "123 main street",
"city": "New York",
"localityCode": "NY",
"zipCode": "10001",
"countryCode": "USA"
},
"merchantReference": "YOUR MERCHANT REFERENCE",
"merchantId": "YOUR MERCHANT ID",
"shippingOption": "Selected shipping option ID",
"discountCode": "discount code entered by shopper" // parameter will not be sent if shopper does not enter a code
}
}
{
"shippingOptions": [
{
"optionType": "FLAT",
"id": "fast-option-id",
"optionName": "Fast Shipping",
"defaultFee": {
"value": 800,
"uom": "USD"
}
},
{
"optionType": "FLAT",
"id": "free-option-id",
"optionName": "Free Shipping",
"defaultFee": {
"value": 0,
"uom": "USD"
}
}
],
"taxDetails": {
"value": 200,
"uom": "USD"
},
"discountAmount": {
"value": 1000,
"uom": "USD"
}
}
Request Parameters
For this event the request parameters are the standard parameters along with the following
Parameter Name | Type | Description |
---|---|---|
cart | cart object | This will be the cart items |
shippingAddress | shippingAddress object | The shipping address details Example: { "firstName": "John", "lastName": "Doe", "address1": "123 Main St", "address2": "Apt 1", "city": "San Francisco", "localityCode":"CA", "zipCode": "94105", "countryCode":"US" } |
shippingOptions | shippingOptions array | An array of Shipping details |
taxDetails | taxDetails object | An object of Tax details |
discountCode | String | This is the discount code that the user inputs on the Skipify experience if applicable. |
Cart Object Parameters
Parameter Name | Type | Required | Description |
---|---|---|---|
title | string | yes | The title of the item(s) to be displayed |
itemId | string | yes | This is the ID of your line item |
productType | string | no | The type of product. Example: shirt, pizza |
quantity | integer | yes | Line item quantity |
description | string | no | Describes the cart item |
price | unit object | yes | These are the amounts for each product / item |
image | price object | no | {url:"", alt:""} |
ShippingAddress Object Parameters
Parameter Name | Type | Description |
---|---|---|
firstname | string | First Name |
lastname | string | Last Name |
address1 | string | Street address |
address2 | string | Street address |
city | string | City name |
localityCode | string | Locality code could be state, region, territory, province, etc... |
zipCode | string | Zip code |
countryCode | string | Country Code |
ShippingOptions Object Parameters
Parameter Name | Type | Required | Description |
---|---|---|---|
id | string | yes | The unique ID of the shipping method |
optionName | string | yes | The name associated with the shipping method to be displayed Example: USPS Ground, UPS Express, etc |
defaultFee | object | yes | The price associated with the shipping method |
optionType | string | yes | ‘FLAT’, ‘PERITEM’, ‘WEIGHT_RANGE’, ‘PRICE_RANGE’ |
optionDescription | string | no | The description of the shipping method |
TaxDetails Object Parameters
Parameter Name | Type | Description |
---|---|---|
value | integer | The value of an item. The last two digits are cents, so $20.50 would be sent as 2050. |
uom | string | Three letter code to identify the currency. Skipify is only processing transactions in USD at this time. Make sure you are passing USD as a value. |
Response Parameters
Parameter Name | Type | Description |
---|---|---|
cart | cart object | This will be the cart items |
shippingAddress | shippingAddress object | The shipping address details |
shippingOptions | shippingOptions object | An array of Shipping details |
taxDetails | taxDetails object | An object of Tax details |
discountAmount | discountAmount object | This is the discount value |
Cart Object Parameters
Parameter Name | Type | Required | Description |
---|---|---|---|
title | string | yes | The title of the item(s) to be displayed |
itemId | string | yes | This is the ID of your line item |
productType | string | no | The type of product. Example: shirt, pizza |
quantity | integer | yes | Line item quantity |
description | string | no | Describes the cart item |
price | unit object | yes | These are the amounts for each product / item |
image | price object | no | {url:"", alt:""} |
ShippingAddress Object Parameters
Parameter Name | Type | Description |
---|---|---|
firstname | string | First Name |
lastname | string | Last Name |
address1 | string | Street address |
address2 | string | Street address |
city | string | City name |
localityCode | string | Locality code could be state, region, territory, province, etc... |
zipCode | string | Zip code |
countryCode | string | Country Code |
ShippingOptions Object Parameters
Parameter Name | Type | Required | Description |
---|---|---|---|
id | string | yes | The unique ID of the shipping method |
optionName | string | yes | The name associated with the shipping method to be displayed Example: USPS Ground, UPS Express, etc |
defaultFee | object | yes | The price associated with the shipping method |
optionType | string | yes | ‘FLAT’, ‘PERITEM’, ‘WEIGHT_RANGE’, ‘PRICE_RANGE’ |
optionDescription | string | no | The description of the shipping method |
TaxDetails Object Parameters
Parameter Name | Type | Description |
---|---|---|
value | integer | The value of an item. The last two digits are cents, so $20.50 would be sent as 2050. |
uom | string | Three letter code to identify the currency. Skipify is only processing transactions in USD at this time. Make sure you are passing USD as a value. |
DiscountAmount Array
Parameter Name | Type | Required | Description |
---|---|---|---|
value | integer | yes | The amount of the Discount for the discountCode applied for this order (if applicable). The last two digits are cents, so $20.50 would be sent as 2050. |
uom | string | yes | Three letter code to identify the currency. Skipify is only processing transactions in USD at this time. Make sure you are passing USD as a value. |
ORDER_VERIFY Callback Event
This callback event is used to validate the order details before a payment is processed. It’s an optional additional check you can use to ensure the order can be completed before the payment is authorized. This is helpful to use with rapidly changing inventory or order lifecycle changes.
Request Parameters
Skipify will pass the order information compiled from other events including product information, shipping address, pricing information, and if applicable, shipping options and discount codes.
Sample ORDER_VERIFY Request and Response
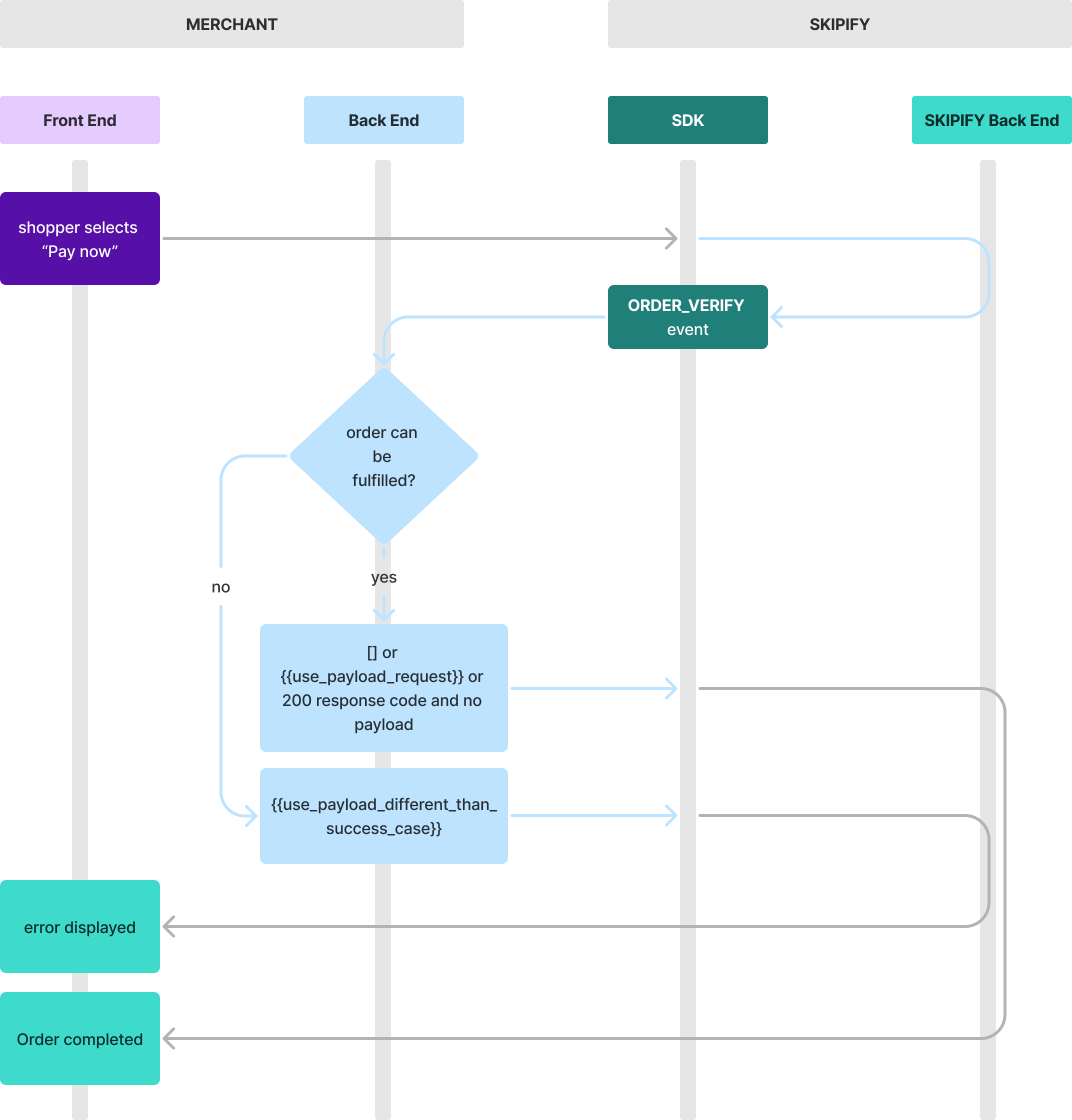
{
"merchantReference": "YOUR MERCHANT REFERENCE",
"merchantId": "YOUR MERCHANT ID",
"eventName": "ORDER_VERIFY",
"eventType": "sync",
"live": false,
"cart": [], // your cart details here
"shippingAddress": {
"firstName": "String",
"lastName": "String",
"address1": "String",
"address2": "String",
"city": "String",
"localityCode": "String",
"zipCode": "String",
"countryCode": "String"
},
"shippingOptions": [
{
"id": "String",
"optionName": "String",
"optionType": "String",
"defaultFee": {
"value": 1000, // integer
"uom": "USD"
}
}
],
"taxDetails": [
{
"systemOfOriginId": "String",
"value": 500, // integer
"uom": "USD",
"code": "String"
}
],
"discountAmount": {
"value": 0, // integer
"uom": "USD"
}
}
Skipify needs to know if the order information passed in the request can be fulfilled.
Valid responses could be:
- An empty array that signifies the order can be fulfilled
- Payload including the same properties back
- 200 response code w/o response payload
Response Parameters
Note: An error produced during this step means that the order can not be fulfilled. Skipify will prompt the user to make adjustments to the order before triggering this callback again.
Front End Callbacks (optional)
The purpose of these callbacks is to provide data for merchants to create customized logic handling when certain events occur, such as closing a component or after a successful transaction.
Implementation Details:
The implementation involves utilizing two or three callback functions: onClose
, onApprove
and onClick
. These functions are passed as options to the button
method provided by the Skipify Checkout SDK.
The email
method only utilizes the onClose
and onApprove
functions.
Below is the structure of the options object and example usages:
const options = {
onClose: (myRef: string, success: boolean) => { // optional
// Your onClose callback code here
console.log('On close UI callback triggered')
},
onApprove: (myRef: string, data: any) => { // optional
// Your onApprove callback code here
console.log('On approve UI callback triggered')
console.log(data)
}
};
skipifySdk.email("your merchant reference here", options).enable(<your-input-object>);
const options = {
onClose: (myRef, success) => { //optional
// Your onClose callback code here
},
onApprove: (myRef, data) => { //optional
// Your onApprove callback code here
},
onClick: (myRef) => { //optional
//Your onClick callback code here
};
skipifySdk.button("your-merchantReference", options).render( < your-container > );
These callbacks accept parameters that provide context about the event:
myRef
: A string parameter representing your unique merchant identifier or reference associated with the transaction.
success
: A boolean parameter indicating the success or failure of the transaction (applicable for onClose callback).
data
: An object containing additional data or payload associated with the action (applicable for onApprove callback).
{
"merchantReference": "6695419b6ccfeb6b7046b3c9",
"customerInfo": {
"email": "[email protected]",
"paymentMethod": {
"cardBrand": "visa",
"expMonth": 12,
"expYear": 29,
"fullNameCard": "Test Person",
"last4CardNumber": "1111"
},
"phoneNumber": "2604250218"
},
"fees": [
{
"type": "general",
"value": 500,
"uom": "USD"
},
{
"type": "surcharge",
"value": 289,
"uom": "USD"
}
],
"shippingAddress": {
"address1": "123 1/2 Duboce Ave",
"address2": null,
"city": "San Francisco",
"countryCode": "US",
"firstName": "Test",
"lastName": "Person",
"localityCode": "CA",
"zipCode": "94103"
},
"billingAddress": {
"address1": "123 1/2 Duboce Ave",
"address2": null,
"city": "San Francisco",
"countryCode": "US",
"firstName": "Test",
"lastName": "Person",
"phoneNumber": "2604250218",
"localityCode": "CA",
"zipCode": "94103"
},
"shippingOptions": {
"id": "fast-option-id",
"optionName": "Fast Shipping",
"defaultFee": 800
},
"taxDetails": {
"value": 289,
"uom": "USD"
},
"tipAmount": {
"value": 400,
"uom": "USD"
},
"total": {
"value": 5000,
"uom": "USD"
}
}
Callback Implementation
onClose Callback:
- Use the
onClose
callback to define actions to be taken when the component is closed. - Utilize the
myRef
parameter to identify the specific component or action associated with the closure. - Optionally, use the
success
parameter to determine the success status of the action.
onApprove Callback:
- Use the
onApprove
callback to define actions to be taken when an action is approved. - Utilize the
myRef
parameter to identify the specific component or action associated with the approval. - Utilize the
data
parameter to access any additional information or payload associated with the approval.
onClick Callback:
- Use the
onClick
callback to track when the Skipify button is clicked if you need to implement a checkout timeout via page refresh - Utilize the
myRef
parameter to identify the specific action associated with the click.
Updated about 1 month ago